if you are searching for a blog on How to connect Node.js to MongoDB then this blog is just for you.
If you’re stepping into the world of backend development with Node.js, it’s essential to have a solid understanding of how to connect your application to databases. One of the most popular choices for databases is MongoDB, a NoSQL database that stores data in a flexible, JSON-like format. In this blog post, we’ll guide you through the process of connecting your Node.js application to MongoDB, step by step.
Table of Contents
- Introduction to MongoDB and Node.js
- Installing MongoDB and Setting Up Your Project
- Connecting to MongoDB using the Official Driver
- Performing CRUD Operations
- 4.1 Creating Data
- 4.2 Reading Data
- 4.3 Updating Data
- 4.4 Deleting Data
- Conclusion
1. Introduction to MongoDB and Node.js
Before we dive into the technical aspects, let’s briefly understand what MongoDB and Node.js are.
MongoDB: MongoDB is a NoSQL database that provides a flexible, schema-less way to store and retrieve data. It’s particularly well-suited for applications with rapidly evolving requirements or complex data structures.
Node.js: Node.js is a runtime environment that allows you to run JavaScript code on the server-side. It’s known for its non-blocking, event-driven architecture, making it perfect for building scalable and efficient backend applications.
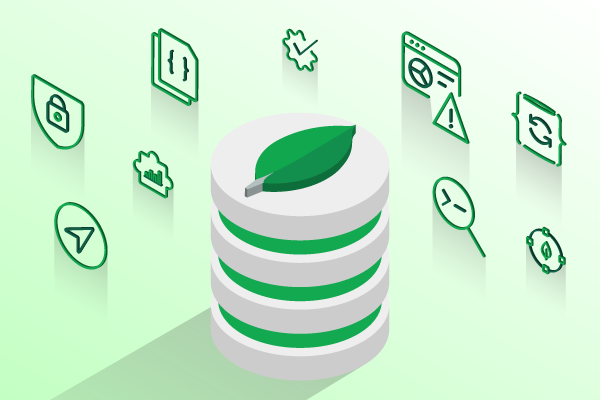
2. Installing MongoDB and Setting Up Your Project
To get started, you need to have Node.js and MongoDB installed on your machine.
- Node.js: Visit the official Node.js website (https://nodejs.org/) and download the LTS version for your operating system. Follow the installation instructions.
- MongoDB: Head to the MongoDB website (https://www.mongodb.com/) and download the Community Server edition. Install it according to the documentation for your operating system.
- Setting Up Your Project: Create a new directory for your project and open a terminal window. Run the following command to initialize a new Node.js project:
npm init -y
This will generate a package.json
file that keeps track of your project’s dependencies and settings.
3. Connecting to MongoDB using the Official Driver
MongoDB offers an official driver for Node.js called mongodb
. To install it, run the following command:
npm install mongodb
Now, let’s establish a connection to your MongoDB database in your Node.js application. Create a file named app.js
(or any other name you prefer) in your project directory.
// Import the MongoDB client
const MongoClient = require('mongodb').MongoClient;
// Connection URL
const url = 'mongodb://localhost:27017';
// Database Name
const dbName = 'myproject';
// Create a new MongoClient
const client = new MongoClient(url, { useNewUrlParser: true, useUnifiedTopology: true });
// Connect to the MongoDB server
client.connect((err) => {
if (err) {
console.error('Error connecting to MongoDB:', err);
return;
}
console.log('Connected successfully to MongoDB server');
// Perform database operations here
// Close the connection
client.close();
});
Replace 'myproject'
with the name of your database. This code establishes a connection to the MongoDB server running locally on the default port (27017).
4. Performing CRUD Operations
Now that you’re connected to MongoDB, let’s perform some basic CRUD (Create, Read, Update, Delete) operations.
4.1 Creating Data
To insert a document into a collection, you can use the insertOne
or insertMany
methods:
// ...
// Select the database
const db = client.db(dbName);
// Select a collection
const collection = db.collection('documents');
// Insert a single document
collection.insertOne({ name: 'John Doe', age: 30 }, (err, result) => {
if (err) {
console.error('Error inserting document:', err);
return;
}
console.log('Document inserted:', result.ops);
});
4.2 Reading Data
To retrieve documents from a collection, you can use the find
method:
// ...
// Find documents
collection.find({ age: { $gt: 25 } }).toArray((err, docs) => {
if (err) {
console.error('Error fetching documents:', err);
return;
}
console.log('Documents found:', docs);
});
4.3 Updating Data
Updating documents is done using the updateOne
or updateMany
methods:
// ...
// Update a document
collection.updateOne({ name: 'John Doe' }, { $set: { age: 31 } }, (err, result) => {
if (err) {
console.error('Error updating document:', err);
return;
}
console.log('Document updated:', result.result);
});
4.4 Deleting Data
Deleting documents can be achieved using the deleteOne
or deleteMany
methods:
// ...
// Delete a document
collection.deleteOne({ name: 'John Doe' }, (err, result) => {
if (err) {
console.error('Error deleting document:', err);
return;
}
console.log('Document deleted:', result.result);
});
5. Conclusion
Congratulations! You’ve learned how to connect your Node.js application to a MongoDB database and perform basic CRUD operations. This is just the beginning of your journey into building robust and scalable backend applications using Node.js and MongoDB. As you gain more experience, you’ll discover advanced topics like indexing, aggregation, and optimization.
Remember, the key to mastering any technology is practice. Experiment with different scenarios, build sample applications and explore the extensive documentation provided by both the Node.js and MongoDB communities. Happy coding!
Also Read: Node JS interview questions with github solutions