[et_pb_section admin_label=”section”]
[et_pb_row admin_label=”row”]
[et_pb_column type=”4_4″][et_pb_text admin_label=”Text”]
NodeJS Interview Questions and Answers
Node.js has become a popular choice for building server-side applications, making it a crucial skill for developers. This blog post will cover some of the most common Node.js interview questions and their answers to help you prepare for your next interview.
1. What is Node.js?
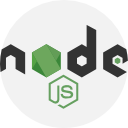
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});
server.listen(3000, '127.0.0.1', () => {
console.log('Server running at http://127.0.0.1:3000/');
});
Node.js is an open-source, cross-platform, JavaScript runtime environment that executes JavaScript code outside a web browser. It allows developers to use JavaScript to write command-line tools and for server-side scripting—running scripts server-side to produce dynamic web page content before the page is sent to the user’s web browser.
2. Why is Node.js Single-threaded?
Node.js is single-threaded to efficiently manage multiple concurrent connections with a non-blocking I/O and an event-driven architecture. This design choice helps Node.js handle thousands of concurrent connections with a single server, unlike traditional web-serving methods that create limited threads to handle requests.
Despite being single-threaded, Node.js can handle multiple requests concurrently. Here’s an example:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('App is listening on port 3000');
});
Even though it’s single-threaded, it can handle any number of requests to the root route concurrently.
3. What is the Event Loop in Node.js?
The event loop in Node.js is a mechanism that allows Node.js to perform non-blocking I/O operations, despite JavaScript being single-threaded. It uses callbacks to signal the completion of a task, enabling the execution of other tasks simultaneously.
console.log('Start');
setTimeout(() => {
console.log('Timeout callback');
}, 0);
Promise.resolve().then(() => {
console.log('Promise resolve');
});
console.log('End');
4. What are Streams in Node.js?
Streams are collections of data, like arrays or strings, but they differ in that they might not be available all at once and don’t have to fit in memory. This makes streams really powerful when working with large amounts of data, or data that’s coming from an external source one chunk at a time.
const fs = require('fs');
const readStream = fs.createReadStream('./largeFile.txt');
readStream.on('data', (chunk) => {
console.log('Received bytes: ', chunk.length);
});
readStream.on('end', () => {
console.log('Read stream finished');
});
5. What is the purpose of module.exports in Node.js?
In Node.js, each file is treated as a separate module. The module.exports or exports is a special object which is included in every JavaScript file in the Node.js application by default. module.exports is used to expose functions, objects or values from the module so they can be used by other programs.
// greet.js
module.exports = function() {
console.log('Hello world');
}
// app.js
const greet = require('./greet');
greet(); // logs 'Hello world'
6. What is the difference between setImmediate() and setTimeout()?
setImmediate() and setTimeout() are both functions of the Timers module in Node.js. setImmediate() is used to execute a script once the current poll phase completes, while setTimeout() schedules a script to be run after a minimum threshold in ms has elapsed.
console.log('start');
setTimeout(() => console.log('setTimeout'), 0);
setImmediate(() => console.log('setImmediate'));
console.log('end');
7. What is a Callback in Node.js?
A callback is a function called at the completion of a given task. This allows other code to be run in the meantime and prevents any blocking. Node.js makes heavy use of callbacks. All the APIs of Node.js are written in such a way that they support callbacks.
const fs = require('fs');
fs.readFile('example.txt', 'utf8', function(err, data) {
if (err) throw err;
console.log(data);
});
8. What is the difference between process.nextTick() and setImmediate()?
process.nextTick() and setImmediate() are part of the Node.js event loop. process.nextTick() takes a callback function and adds it to the “next tick queue” which will be executed on the next iteration of the event loop. setImmediate() executes a callback on the next cycle of the event loop and returns an Immediate object for possible cancellation.
setImmediate(() => console.log('setImmediate'));
process.nextTick(() => console.log('nextTick'));
console.log('end');
//The output will be:
end
nextTick
setImmediate
9. What is the purpose of Buffer class in Node.js?
The Buffer class in Node.js is used to handle binary data. Since JavaScript didn’t initially support binary data, the Buffer class was introduced to Node.js to interact with TCP streams, file system operations, and other contexts that expect binary data.
const buf = Buffer.from('Hello World');
console.log(buf.toString()); // logs 'Hello World'
10. What is the difference between Promise and Async/Await?
Promise and Async/Await are both abstractions for dealing with asynchronous operations. Promise is an object representing the eventual completion or failure of an asynchronous operation. Async/Await is a syntactic sugar on top of Promises, making asynchronous code look and behave like synchronous code.
// Promise
new Promise((resolve, reject) => {
setTimeout(() => resolve('Promise resolved'), 1000);
}).then(console.log);
// Async/Await
(async function() {
const result = await new Promise((resolve, reject) => {
setTimeout(() => resolve('Promise resolved with Async/Await'), 1000);
});
console.log(result);
})();
GitHub Link:
https://gist.github.com/paulfranco/9f88a2879b7b7d88de5d1921aef2093b
https://devinterview.io/dev/nodejs-interview-questions
Conclusion
Node.js is a powerful tool for developing server-side applications. Understanding its core concepts, such as the event loop, streams, modules, and handling asynchronous operations, is crucial for any Node.js developer. This blog post has covered some of the most common Node.js interview questions, and their answers should help you prepare for your next Node.js interview. Good luck!
Also Read: How to use mid-journey for free