Iterating through objects in JavaScript is a fundamental skill for any web developer. Objects in JavaScript are collections of key-value pairs, where each key is unique and associated with a value. Iterating over these key-value pairs allows developers to manipulate, access, and utilize the data stored in objects effectively. This blog will explore various methods to iterate through objects in JavaScript, discussing their syntax, use cases, and best practices.
By the end of this blog, you will learn different ways on How to iterate through an object in JavaScript.
Understanding JavaScript Objects
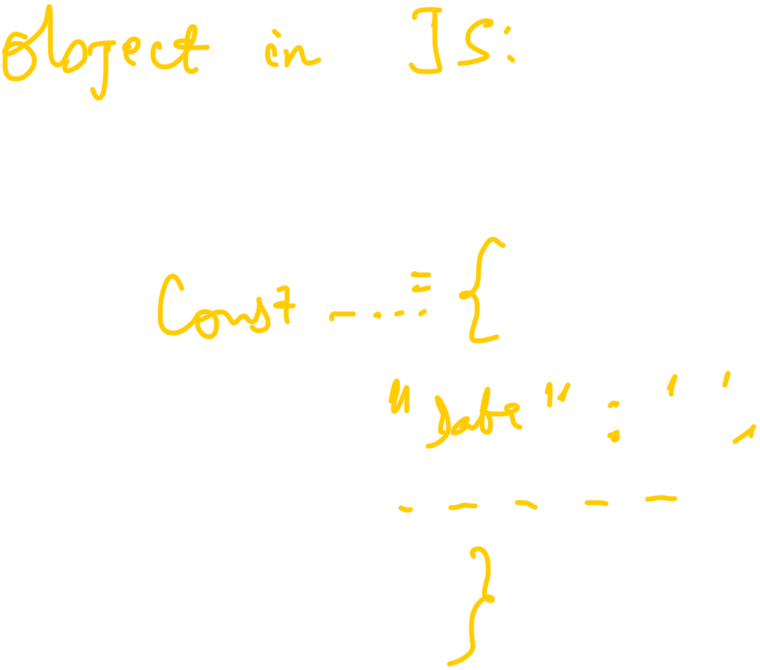
Before diving into iteration, let’s briefly understand what JavaScript objects are. An object in JavaScript can be created using curly braces {}
, with key-value pairs defined within. The keys can be strings or symbols, and the values can be any data type, including numbers, strings, arrays, functions, and even other objects.
const person = {
name: 'John Doe',
age: 30,
occupation: 'Web Developer'
};
In this example, person
is an object with three properties: name
, age
, and occupation
.
for…in Loop
The for...in
loop is one of the most straightforward methods to on how to iterate over an object’s properties in JavaScript. It loops through the properties of an object, allowing you to execute a block of code for each property.
for (let key in person) {
console.log(key + ': ' + person[key]);
}
This loop will log each property name and its value to the console. However, it’s important to note that for...in
will also iterate over properties inherited through the prototype chain. To ensure you only iterate over the object’s own properties, use Object.hasOwnProperty()
:
for (let key in person) {
if (person.hasOwnProperty(key)) {
console.log(key + ': ' + person[key]);
}
}
Object.keys(), Object.values(), and Object.entries()
ECMAScript 5 introduced several methods to work with objects more efficiently: Object.keys()
, Object.values()
, and Object.entries()
.
Object.keys(obj)
returns an array of an object’s own property names.Object.values(obj)
returns an array of an object’s own enumerable property values.Object.entries(obj)
returns an array of an object’s own enumerable string-keyed property[key, value]
pairs.
Using Object.keys()
:
Object.keys(person).forEach(key => {
console.log(key + ': ' + person[key]);
});
Using Object.values()
:
Object.values(person).forEach(value => {
console.log(value);
});
Using Object.entries()
:
Object.entries(person).forEach(([key, value]) => {
console.log(key + ': ' + value);
});
These methods make it easier to iterate over objects without worrying about inherited properties.
Using for…of with Object.entries()
Combining for...of
loop with Object.entries()
provides a clean and modern way to iterate through an object’s properties:
for (let [key, value] of Object.entries(person)) {
console.log(key + ': ' + value);
}
This approach is both concise and readable, making it a popular choice among developers.
Higher-Order Functions
JavaScript’s array higher-order functions, such as forEach()
, map()
, filter()
, and reduce()
, can be utilized with Object.keys()
, Object.values()
, and Object.entries()
to perform more complex operations while iterating over objects.
For example, to create an array of strings that contain both the key and value of each property in the person
object:
const keyValuePairs = Object.entries(person).map(([key, value]) => `${key}: ${value}`);
console.log(keyValuePairs);
Conclusion
Iterating through objects in JavaScript is a common task, and understanding the various methods available can significantly enhance your coding efficiency and capability. Whether you choose the traditional for...in
loop or the more modern methods like Object.entries()
with for...of
, the best choice depends on your specific use case and the requirements of your project.
As you continue to learn and build projects with JavaScript, experiment with these different iteration techniques to find which ones best suit your coding style and project needs. Remember, the best way to learn is by doing, so consider applying these methods in your next project or coding challenge.