[et_pb_section fb_built=”1″ admin_label=”section” _builder_version=”3.22″][et_pb_row admin_label=”row” _builder_version=”3.25″ background_size=”initial” background_position=”top_left” background_repeat=”repeat”][et_pb_column type=”4_4″ _builder_version=”3.25″ custom_padding=”|||” custom_padding__hover=”|||”][et_pb_text admin_label=”Text” _builder_version=”3.27.4″ background_size=”initial” background_position=”top_left” background_repeat=”repeat”]
Asynchronous Programming in javascript is simply done with callbacks.
Asynchronous programming uses multiple tasks running in parallel with no delay between them. This makes any application faster because waiting on UI events stops its performance from dropping. It’s also easier to manage as you don’t have to wait for the entire program to finish before proceeding. This type of programming is best for applications that need to respond quickly to user input and produce a result over time. Examples include games and real-time communication tools such as Discord and TeamSpeak. In contrast, synchronous programming waits for all of the code in a program to finish before moving on. This limits how fast your app can respond to user input, but it’s safer and more secure since waiting won’t corrupt anything you do later on.
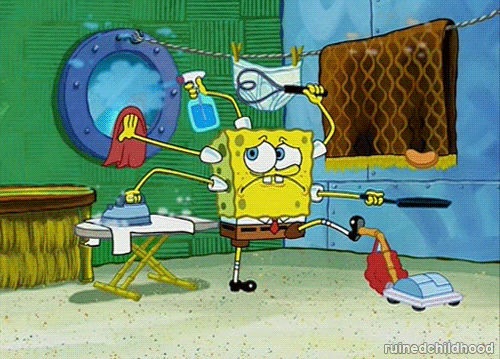
One of the biggest advantages of using asynchronous programming is how much better it makes web applications feel. Waiting for events to happen slows down every part of an app’s performance when used with UI elements like mouse or touch events.
Async in NodeJS implements this programming style, making web apps run significantly faster than their non-Async counterparts without sacrificing security or responsiveness.
3 Things to know in Asynchronous in Javascript:
- Promises (new in ES6)
- Async Await which was introduced in 2017
- For /await loop added in 2018
Defining your own custom promises is easy if you understand how asynchronous coding works behind the scenes— there are no delays involved once you understand that part since you control exactly where delays occur or don’t with custom promises instead. Customizing how things work behind the scenes lets you create whatever result you want by defining what happens when certain conditions are met or not met by your promise chain’s endpoints. For example, setting a timeout on your promise chain will stop it after that amount of time has passed without producing any result unless someone performs an action or resolves another promise within that time frame first.
Event-driven in Javascript
In Javascript, Client-side programs are almost event-driven which means any task required a user to do something and then respond to the user’s actions.
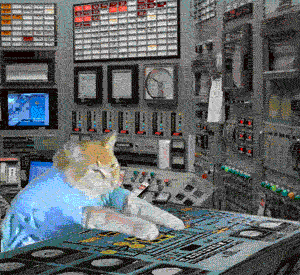
For Example, Whenever a click of the mouse or a button on the keyboard is pressed, event-driven javascript programs register callback functions for specific types of events in specified contexts the web browser invokes the function wherever the specific events occur. These callbacks are called event handlers or event listeners who listen to a specific type of event.
In Js, event listner is registered with addEventListener();
Promise in JavaScript
A promise is an object that represents the result of an asynchronous operation.
It must be fulfilled or rejected. A promise can never be both.
Promises are a great way to write javascript when compared with traditional asynchronous coding techniques since they produce results instantly and enhance performance at the same time— especially when compared with Define Own Custom Promises! It can be challenging to get started with asynchronous coding at first since things look so similar after gaining experience with synchronous programs for so long now! However, once you understand how asynchronous programming works and what its benefits are, implementing this into your projects becomes easy! Asynchronous programs run much smoother and enhance user experience much more than traditional synchronous programs ever could!
Simple Example of Promise in JS:
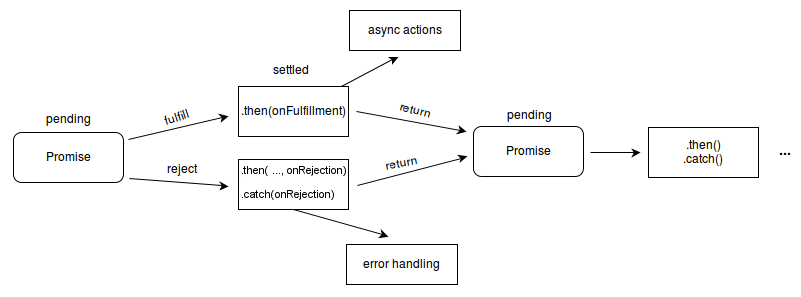
myPromise.then(function (result) {
// Resolve callback.
console.log(result);
console.log('test');
}, function (result) {
// Reject callback.
console.error(result);
});
Async Await in JavaScript
Example of an async await in js:
const getUsersDetails = (ms) => { // No need to make this async
return new Promise(resolve => setTimeout(resolve, ms));
};
// this function is async as we need to use await inside it
export const indexData = async (req, res) => {
await getUsersData(5000);
res.json([
{
id: 1,
name: 'John Doe',
},
{ id: 2,
name: 'Jane Doe',
},
]);
};
Also Read: 3 Best File Sharing Websites in 2022
[/et_pb_text][/et_pb_column][/et_pb_row][/et_pb_section]