This tutorial is all dedicated to loading CSS and javascript dynamically in HTML
Dynamic script injection can be useful in certain scenarios where you need to modify or enhance the behavior of a web page or application at runtime. Here are a few reasons why dynamic script injection may be necessary:
- Dynamic content updates: Injecting scripts dynamically allows you to update the content or behavior of a web page without requiring a full page reload. This can result in a more responsive and interactive user experience.
- Third-party integrations: Many web applications integrate with external services or APIs that require the use of JavaScript. Dynamic script injection enables the insertion of scripts from these third-party sources, allowing seamless integration with external functionality.
- Customization and personalization: By injecting scripts dynamically, you can tailor the user experience based on specific conditions or user preferences. This could include modifying the appearance of elements, adding interactive features, or adapting the content based on user inputs.
- A/B testing and analytics: Dynamic script injection is often used for A/B testing, where different versions of a web page are served to different users. It allows you to inject specific tracking scripts to measure user interactions, gather analytics data, and analyze user behavior.
- Security enhancements: In certain cases, dynamic script injection can be used for security-related purposes. For example, injecting scripts to implement additional client-side validation or security measures, such as input sanitization or CSRF protection.
It’s important to note that dynamic script injection should be used judiciously and with caution, as it can introduce security risks, such as cross-site scripting (XSS) vulnerabilities, if not implemented properly. Careful consideration should be given to input validation, output encoding, and other security best practices to mitigate these risks.
Web development is a dynamic field that requires constant learning and adaptation. One of the most useful techniques in modern web development is the dynamic loading of CSS and JavaScript files in HTML. This technique allows developers to load resources on demand, improving the performance and user experience of web applications. This article will guide you through the process of dynamically loading CSS and JavaScript files in HTML.
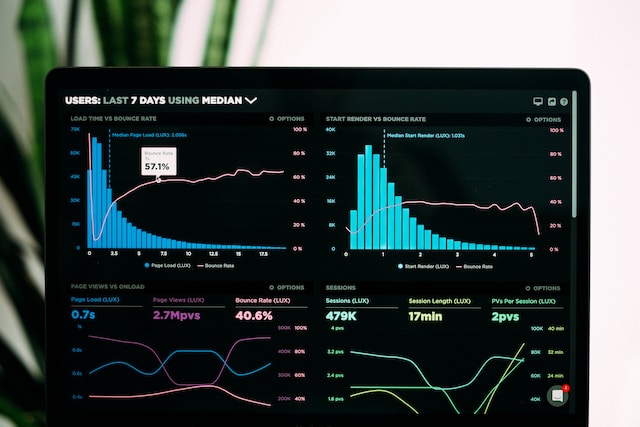
Understanding Dynamic Loading
Before we delve into the how, it’s important to understand the why. Dynamic loading is a technique that allows a program to load resources during runtime, as opposed to loading all resources at the start of the program. This can significantly improve the performance of your web application, especially if it relies on large CSS and JavaScript files.
Dynamically Loading CSS
To dynamically load a CSS file, you need to create a new link element, set its rel attribute to “stylesheet”, its href attribute to the URL of the CSS file, and then append this element to the head of the document. Here’s a simple JavaScript function that does this:
function loadCSS(url) {
var link = document.createElement("link");
link.rel = "stylesheet";
link.href = url;
document.head.appendChild(link);
}
You can call this function with the URL of the CSS file you want to load:
loadCSS("styles.css");
Dynamically Loading JavaScript
Loading a JavaScript file dynamically is similar to loading a CSS file. You create a new script element, set its src attribute to the URL of the JavaScript file, and then append this element to the body of the document. Here’s a JavaScript function that does this:
function loadJS(url) {
var script = document.createElement("script");
script.src = url;
document.body.appendChild(script);
}
You can call this function with the URL of the JavaScript file you want to load:
loadJS("script.js");
Handling Load Events
When you load a CSS or JavaScript file dynamically, you might want to know when the file has finished loading so you can use the styles or functions defined in it. You can do this by listening to the load event of the link or script element.
Here’s how you can modify the loadCSS and loadJS functions to accept a callback function that is called when the CSS or JavaScript file has finished loading:
function loadCSS(url, callback) {
var link = document.createElement("link");
link.rel = "stylesheet";
link.href = url;
link.onload = callback;
document.head.appendChild(link);
}
function loadJS(url, callback) {
var script = document.createElement("script");
script.src = url;
script.onload = callback;
document.body.appendChild(script);
}
You can use these functions like this:
loadCSS("styles.css", function() {
console.log("CSS has loaded!");
});
loadJS("script.js", function() {
console.log("JavaScript has loaded!");
});
Conclusion
Dynamic loading of CSS and JavaScript files is a powerful technique that can significantly improve the performance of your web applications. By loading resources on demand, you can reduce the initial load time of your application and provide a better user experience. The JavaScript functions presented in this article provide a simple way to dynamically load CSS and JavaScript files in HTML. By understanding and using this technique, you can take your web development skills to the next level.
Also Read: First Class Function in JavaScript