DOM stands for Document Object Model, With javascript, we can manipulate content displayed on a site.
Let’s understand more in this article.
As per World Wide Web Consortium commonly known as W3C, DOM defines a standard way to define locate and access documents on the web.
In general, DOM can be categorized into 3 parts:
1) Core DOM
– Basic for all documents
2) XML DOM
– standard for XML Document
3) HTML DOM
– used to access, locate and manipulate HTML Tags and elements
Usually, we can see HTML DOM manipulation in daily life.
The hierarchical structure of DOM
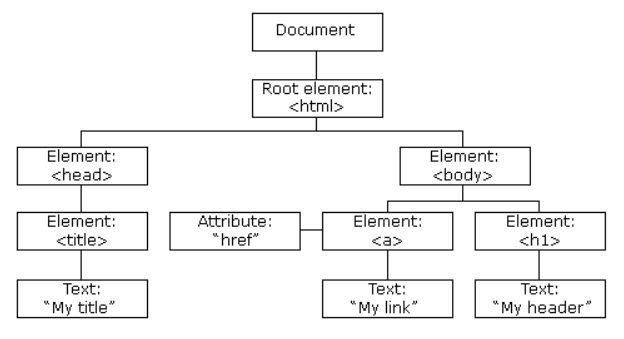
Some of the examples of DOM Manipulation are as follows:
1. Form Validation
When forms submit on-site, with the help of DOM manipulation we can know that all the required entries can be filled out correctly.
2. Dynamic Content Loading
Some sites use dynamic rendering to load content without doing a refresh.
3. UI Tweaks
Websites use widgets like Scroll to top to get the above fold of the site, dark mode, etc are some of the UI changes can be done with DOM manipulation.
Selecting elements
Javascript offers a wide variety of methods to access elements.
Some of the examples are:
getElementById()
getElementsByClassName()
getElementsByName()
getElementsByTagName()
querySelector()
- getElementById() ~ Select an element by Id
- getElementsByClassName() ~ Select an element by classname
- getElementsByName() ~ Select an element by name
- getElementsByTagName() ~ Select an element by Tag name
- querySelector() ~ Select elements by its CSS selector
Let’s understand getElementById() with one example
getElementById()
We need to manipulate elements through DOM so, At First, we need HTML, let’s suppose we have an HTML file like:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JS getElementById() Example</title>
</head>
<body>
<p id="iAmTheId">This is a demo paragraph file</p>
</body>
</html>
below is the <p> tag with the attribute iAmTheId. Later the id value will be used to manipulate the elements
<p id="iAmTheId">This is a demo paragraph file</p>
Now it’s time to use the id value, we will change and log the new value
//Script.js code
var pID = document.getElementById("iAmTheId");
pID.innerHTML = "This is the new text";
Output

You learn more about selectors from the Mozilla Developers Network(MDN resources).
Extra Perk: With the help of a developer tool you can actually log the clickable elements directly into the console.
Open the dev console using the shortcut Ctrl+Shift+J or via right-click inspect.
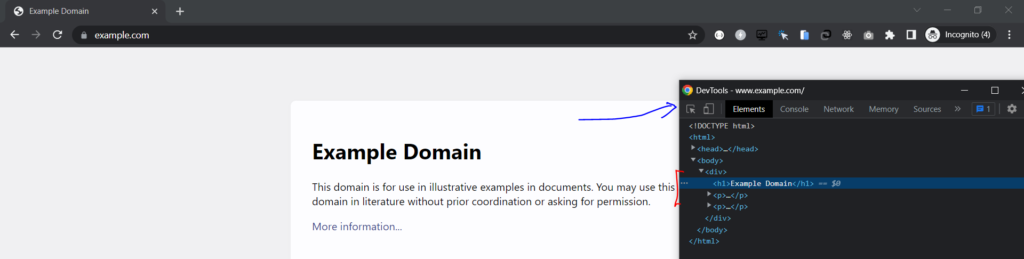
You can print the same value in the console using:
$0
You can even change the value directly from the console using the below code:
$0.innerHTML ="Hello from Tronlab.in"
'Hello from Tronlab.in'
Basically we are accessing the value of the tag through the DOM node and changing its attribute to Hello from tronlab.in
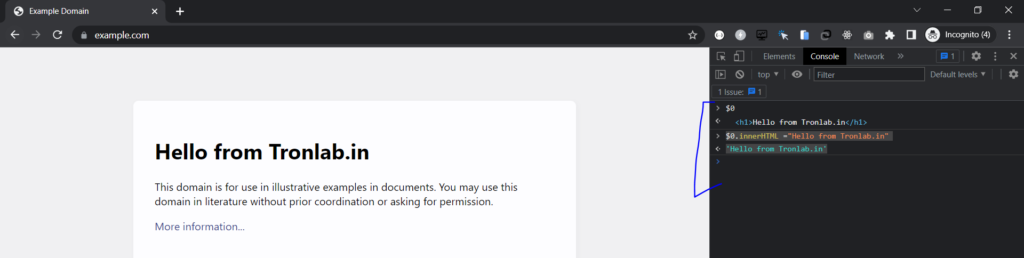
DOM Manipulation game in JavaScript
There are many open-source games made with HTML, CSS, and Javascript.
By adding structure through HTML, style through CSS,
and dom and logic implementation through JS, you can also pretty much make a working game.
With that said, we’ve added a list of games implemented with dom and js:
1. Flappy bird
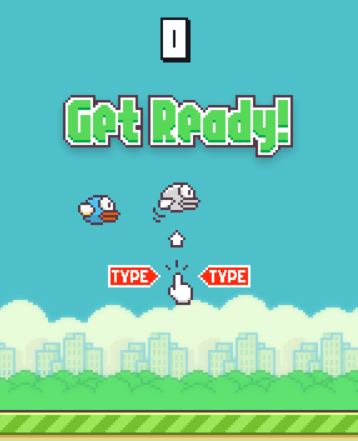
Flappy bird game was originally made in 2014 by Vietnamese programmer Dong Nguyen.
The games remind old-school vibes, if you remember it,
It can still be played, in fact, you can make one by yourself.
Github repo: Flappy bird GitHub repo
2. Game made using HTML, CSS and Javascript
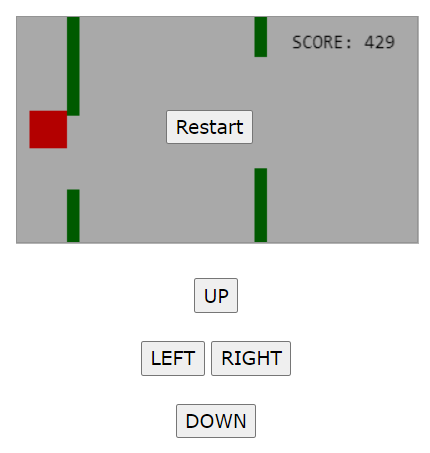
There is one game similar to flappy bird hosted on w3school, you can actually code and get player UI side by side.
The game uses plain HTML, CSS, and Js.
View Project: https://www.w3schools.com/graphics/game_intro.asp
Conclusion
Learning DOM is a must skill to know especially if you are interested in frontend development.
No matter if you beginner, intermediate or advanced coder, DOM in JS help you get an understanding of elements and node linked to each other. With the help, you can make software that can add, remove or alter elements using different methods of dom.
Additionally, you can embed a whole application by just adding the script to any page.
Javascript methods like document.createElement() add elements.
Below is one of example embedding div into a website.
let newDivElement = document.createElement("div");
newDivElement.innerHTML = "Hello, World!";
newDivElement.classList.add("Test");
document.body.appendChild(newDivElement);
Output:
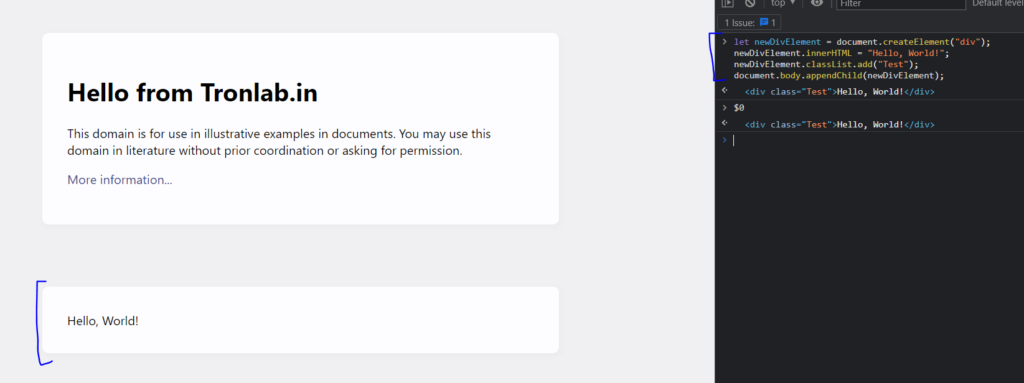
you get the idea, Learning DOM is fundamental to frontend development.
Also Read: The Best Windows hacks that you know