No doubt! React is one of the most loved and widely used libraries in Javascript.
In this article, you will learn to make a random quote generator using React. Moreover, you will get hands-on, file structure, jsx, state management, etc.
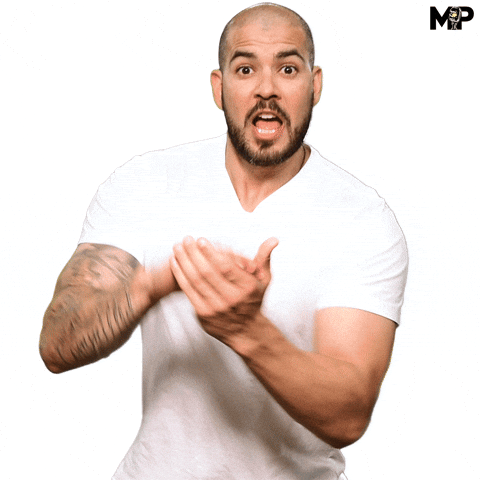
Learning React 18 in 2023 is must skill you should know.
Let’s start with
What are React JS and its file structure
React js is a powerful library written in javascript that offers tons of functionality used to make the application user interface.
React js offers rich documentation and you can read it here, React Documentation.
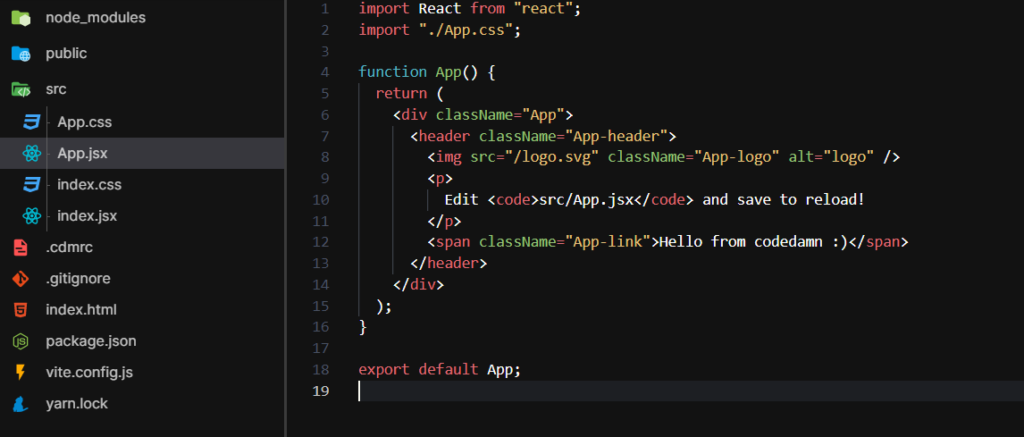
State Management in React with Example
React State management is a way through which react js manages the data of the component when it needs to render it.
In simple terms, it saves the data in state and when that state is called the UI renders the data.
Some of the examples useState hook to manage local states like form submission, toggle dark mode, etc.
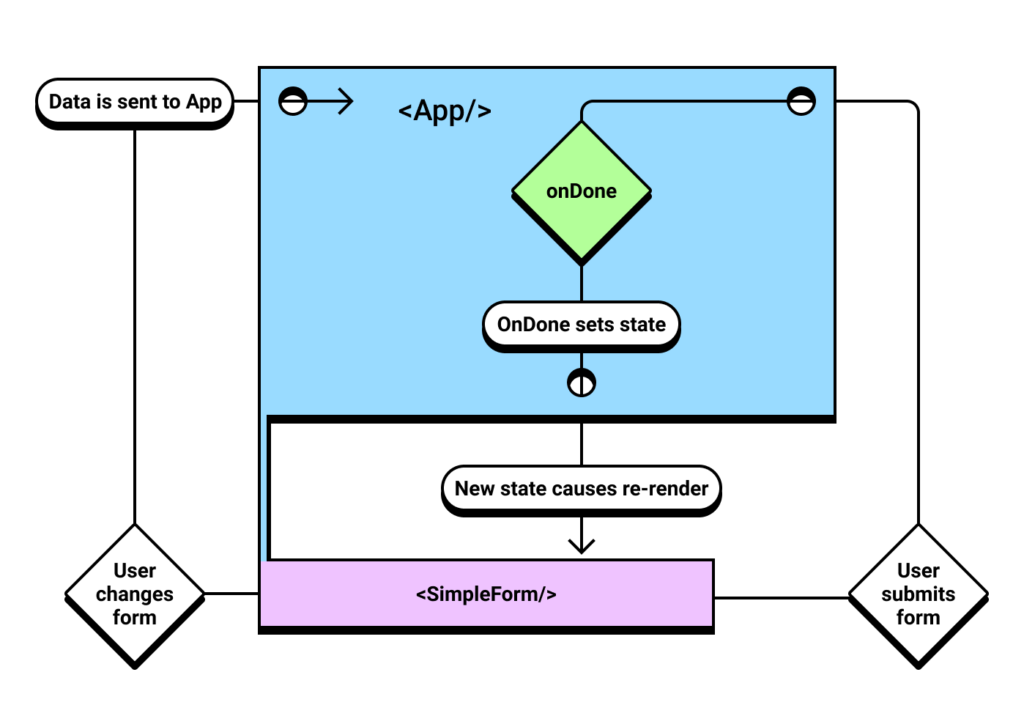
Quote generator made in React JS
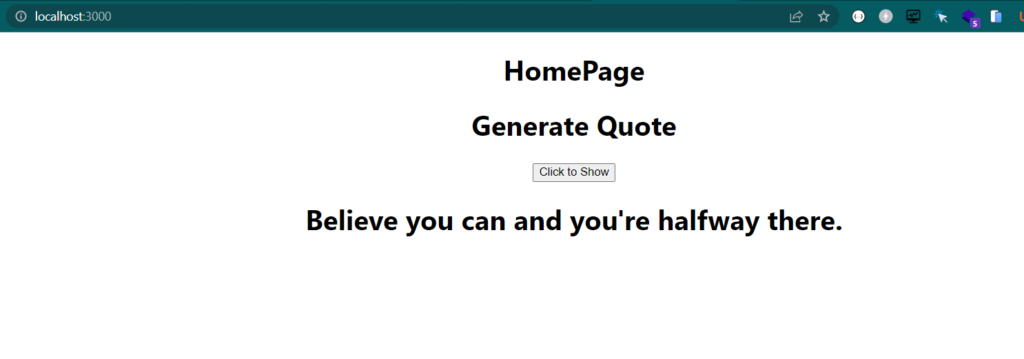
Project Overview:
React Quote Generator offers a new quote every time when clicked on click to show button. The idea is simple: Generate quotes on Click.
Additionally, at the end of this tutorial, you will also get a GitHub repository where you can learn from the code or clone the entire repo to get an understanding.
Without further due, Let’s build react quote application.
Below are the simple steps to build a quote generator:
Quick Note: The goal of this project is to make you help in understanding react state, css is not applied in this project, you can add it by yourself and do share the revamped version of it. We were happy to see that.
Step 1: Make a folder 📂 ReactApp and generate react boilerplate via:
npx create-react-app my-app
cd my-app
npm start
The first command shown above is to initialize the react app setup.
After that, the change directory or short form cd my-app is used to get into the folder where react application is located, i.e my-app.
The code npm start uses to initialize the server.
Step 2: Add a new component for feature,i.e Appfeature.js as shown below
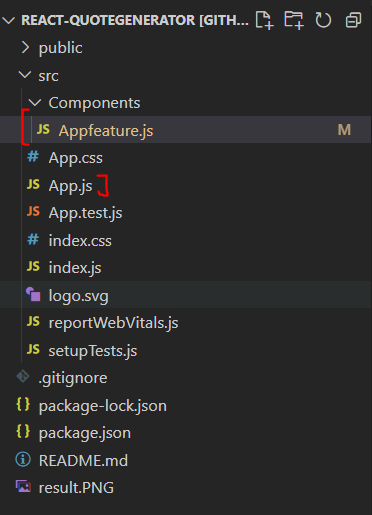
import React from 'react'
const AppFeature = function appFeature(props){
//make a function that will copy to clipboard in react
return(
<>
<h1>{props.feature}</h1>
</>
)
}
export default AppFeature;
Step 3: Add the below code in App.js
import React, {useState} from 'react';
import './App.css';
import AppFeature from './Components/Appfeature';
//array of quotes
// const quoteData = ["Jai Shree Ram","hindu","Abc"]
const quoteData = ["Jai Shree Ram","Believe you can and you're halfway there.", "The only limit to our realization of tomorrow will be our doubts of today.", "Don't watch the clock; do what it does. Keep going.", "It does not matter how slowly you go as long as you do not stop.", "The only way to do great work is to love what you do.", "The greatest glory in living lies not in never falling, but in rising every time we fall.", "There is no substitute for hard work.", "The only limit to our future is the doubts of today.", "Believe in yourself and all that you are. Know that there is something inside you that is greater than any obstacle.", "If you look at what you have in life, you'll always have more. If you look at what you don't have in life, you'll never have enough.", "The only thing stopping you from reaching your goals is yourself.", "If you can dream it, you can do it.", "You are never too old to set another goal or to dream a new dream.", "Success is not the key to happiness. Happiness is the key to success. If you love what you are doing, you will be successful.", "Don't be pushed around by the fears in your mind. Be led by the dreams in your heart.", "The secret of getting ahead is getting started.", "It always seems impossible until it's done.", "You miss 100% of the shots you don't take.", "Hardships often prepare ordinary people for an extraordinary destiny."]
function App() {
//make state
const[quote, setQuote] = useState(quoteData[0]);
//func to radomize Quote
function quoteRandomizer(){
const quoteRandom = quoteData[Math.floor(Math.random() * quoteData.length)]
setQuote(quoteRandom)
}
return (
<div className="App">
<h1>HomePage</h1>
<AppFeature feature={"Generate Quote"}/>
<button onClick={quoteRandomizer}>Click to Show</button>
<h1>{quote}</h1>
</div>
);
}
export default App;
As from the above Appfeature.js component you can new features to your app, the goal to make separate components folder is to get new features to your app.
App.js
In App.js we’ve imported our components and state
//react and it's state
import React, {useState} from 'react';
import './App.css';
//imported component for App features
import AppFeature from './Components/Appfeature';
After that, we added an array where all the quotes are stored, then used the state and functions to store and randomize the data, once that is done we used the onClick event listener in react to show the data.
Conclusion
React state and its functionality is used to show in this blog. The data is stored in react state and every time an action triggers like an onClick event it saves and updates and re-renders the UI to show the changes.
The quote generator is one of the simple projects to get you started with state and functions in React JS.
We hope this article helped you if you’ve any confusion you can write to us.
Project Githu Repo: React Quote Generator Github Repository
Also Read: Windows hidden hacks